These are two simple functions I built for 256-bit encryption/decryption with mcrypt. I've decided to use MCRYPT_RIJNDAEL_128 because it's AES-compliant, and MCRYPT_MODE_CBC. (ECB mode is inadequate for many purposes because it does not use an IV.)
This function stores a hash of the data to verify that the data was decrypted successfully, but this could be easily removed if necessary.
<?php
function encrypt($decrypted, $password, $salt='!kQm*fF3pXe1Kbm%9') {
// Build a 256-bit $key which is a SHA256 hash of $salt and $password.
$key = hash('SHA256', $salt . $password, true);
// Build $iv and $iv_base64. We use a block size of 128 bits (AES compliant) and CBC mode. (Note: ECB mode is inadequate as IV is not used.)
srand(); $iv = mcrypt_create_iv(mcrypt_get_iv_size(MCRYPT_RIJNDAEL_128, MCRYPT_MODE_CBC), MCRYPT_RAND);
if (strlen($iv_base64 = rtrim(base64_encode($iv), '=')) != 22) return false;
// Encrypt $decrypted and an MD5 of $decrypted using $key. MD5 is fine to use here because it's just to verify successful decryption.
$encrypted = base64_encode(mcrypt_encrypt(MCRYPT_RIJNDAEL_128, $key, $decrypted . md5($decrypted), MCRYPT_MODE_CBC, $iv));
// We're done!
return $iv_base64 . $encrypted;
}
function decrypt($encrypted, $password, $salt='!kQm*fF3pXe1Kbm%9') {
// Build a 256-bit $key which is a SHA256 hash of $salt and $password.
$key = hash('SHA256', $salt . $password, true);
// Retrieve $iv which is the first 22 characters plus , base64_decoded.
$iv = base64_decode(substr($encrypted, 0, 22) . ');
// Remove $iv from $encrypted.
$encrypted = substr($encrypted, 22);
// Decrypt the data. rtrim won't corrupt the data because the last 32 characters are the md5 hash; thus any 0 character has to be padding.
$decrypted = rtrim(mcrypt_decrypt(MCRYPT_RIJNDAEL_128, $key, base64_decode($encrypted), MCRYPT_MODE_CBC, $iv), '04');
// Retrieve $hash which is the last 32 characters of $decrypted.
$hash = substr($decrypted, -32);
// Remove the last 32 characters from $decrypted.
$decrypted = substr($decrypted, 0, -32);
// Integrity check. If this fails, either the data is corrupted, or the password/salt was incorrect.
if (md5($decrypted) != $hash) return false;
// Yay!
return $decrypted;
}
?>
Sometimes while installing WordPress plugins, you might come across a message to install / enable Mcrypt extension for PHP. Mcrypt is an interface which supports a wide range of block algorithims. It support Algorithims like DES, TripleDES, GOST, OFB etc.
It’s a very common issue that PHP scripts using mcrypt will not work in out of the box installation of PHP. Following are instructions for installation of php-mcrypt on CentOS 7. In order to install php-mcrypt and resolve the issue with mcrypt support in PHP, we’ll first need to enable EPEL repository in our RHEL/CentOS 7 64 Bit install. Problem/Motivation The MCrypt function is deprecated from PHP 7.1. And is removed from PHP 7.2. The the recommended version of this module at the time of this writing (7.x-2.x), has MCrypt as the default. It is proposed to move MCrypt to a separate project in 7.x-3.x at #2554093. In the meantime, it might be better to throw an exception instead of die outright if MCrypt is not available. How to Install the PHP mcrypt Extension In the examples shown, replace 'X.Y' with your app's PHP version (for example, '7.2'). The mcrypt extension is an interface to the mcrypt cryptography library. This extension is useful for allowing PHP code using mcrypt to run on PHP 7.2+.
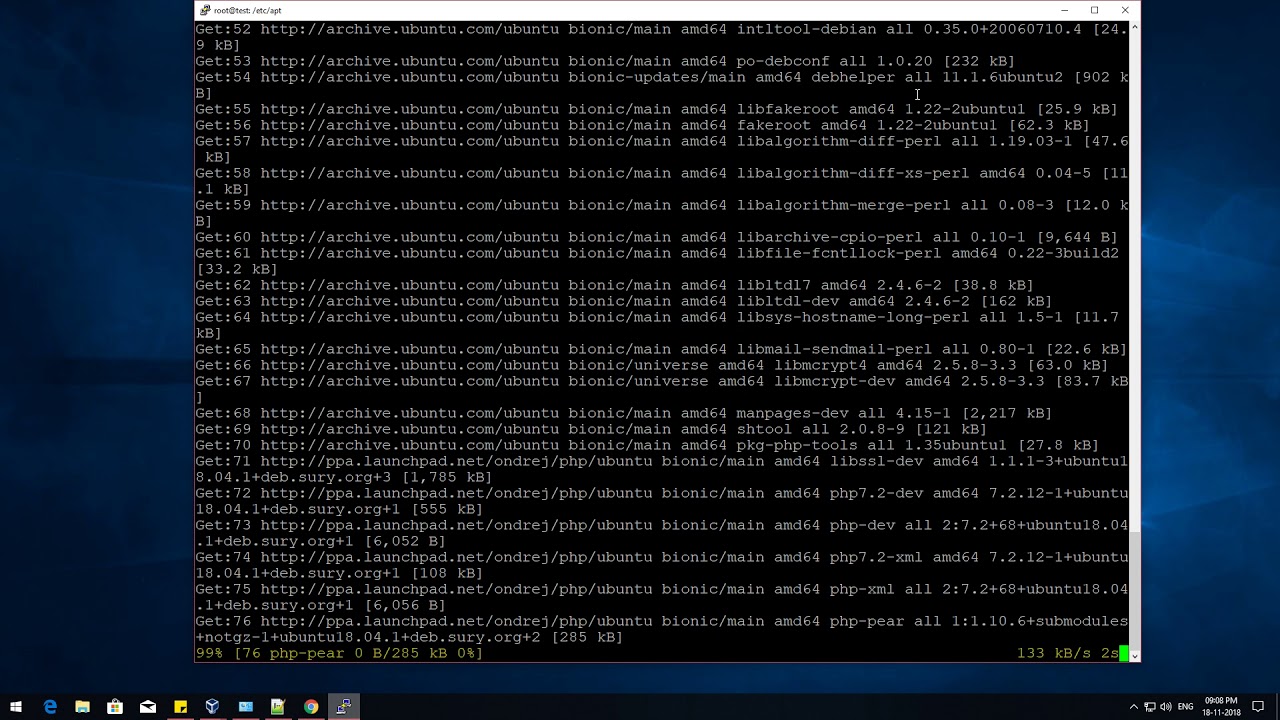
Sometimes while installing WordPress plugins, you might come across a message to install / enable Mcrypt extension for PHP.
Mcrypt is an interface which supports a wide range of block algorithims. It support Algorithims like DES, TripleDES, GOST, OFB etc.
Note : The Mcrypt extension relies on the ‘libmcrypt’ extension, which is not maintained since 2007. So some people consider installing the extension useless. None the less some WordPress plugins do require this extension to be installed and enabled.
Step 1 : Download and isntall the mcrypt extension
Step 2 : Create a symlink for Mcrypt to mods-available
If you are running PHP-FPM your path might be like
Php Mcrypt Install Wamp
If you get an error message like:
This means that the extension is already installed on your server and you just need to enable it by skipping to the next step.
Php Mcrypt Install Android Studio
Step 3 : Enable Mcrypt extension
Php Mcrypt Install Debian
Step 4 Restart PHP and Nginx
